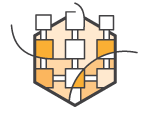


On my spare time I run a website for a tax accounting company. This monolithic app is a largely stateless app (not really but we don’t need persistent stores/volumes), uses one of the best online tax software, and uses ruby on rails and runs on an EC2 instance with Apache and MySQL Server and Client installed. This is what is referenced as a “monolithic app” because all components are installed on 1 single VM. This makes the application more complicated to edit, patch, update etc (even though it barely ever needs to updated apart from making sure the current year’s Tax information and links are updated.) If we want to migrate to a new version of the database or newer version of rails, things are not isolated and can overlap. Now, RVM and other mechanisms can be used to do this, but by using Docker to isolate components and Amazon ECS to deploy the app, we can rapidly develop, and push changes into production in a fraction of the time we used to. This blog post will go through the experience of migrating that “Monolithic” ruby on rails app and converting it into a 2 service Docker application that can be deployed to Amazon ECS using Docker Compose.
First things first:
The first thing I did was ssh into my EC2 instance and figure out what dependencies I had. The following approach was taken to figure this out:
- Use lsb_release -a to see what OS and release we’re using.
- Look at installed packages via “dpkg” / “apt“
- Look at installed Gems used in the Ruby on Rails app, take a peek at Gemfile.lock for this.
- View the history of commands via “history” see any voodoo magic I may have done and forgot about 🙂
- View running processes via “ps [options]” which helped me remember what all is running for this app. E.g. Apache2, MySQL or Postgres, etc.
This gives us a bare minimum of what we need to think about for breaking our small monolith into separate services, at-least from a main “component” viewpoint (e.g. Database and Rails App). It’s more complicated to try and figure out how we can carve up the actual Ra
ils / Ruby code to implement smaller services that make up the site, and in some cases this is where we can go wrong, if it works, don’t break it. Other times, go ahead, break out smaller services and deploy them, but start small, think of it like the process of an amoeba splitting 🙂
We can now move into thinking about the “design” of the app as it applies to microservices and docker containers. Read the next section for more details.
Playing with Legos:
As it was, we had apache server, rails, and mysql all running in the same VM. To move this into an architecture which uses containers, we need to separate some of these services into separate building blocks or “legos” which you could think of as connecting individual legos together to build a single service or app. SOA terms calls this “Composite Apps” which are similar in thinking, less in technology. We’ll keep this simple as stated above and we’re going to break our app into 2 pieces, a MySQL database container and a “Ruby on Rails” container running our app.
Database Container
First, we’ll take a look at how we connect the database with rails. typically in Rails, a connection to a database is configured in a database.yml file and looks something like this. (or something like this)
development:
adapter: mysql
database: AppName_development
username: root
password:
host: localhost
test:
adapter: mysql
database: AppName_test
username: root
password:
host: localhost
production:
adapter: mysql
database: AppName_production
username: root
password:
host: localhost
Now, since we can deploy a MySQL container with Docker using something like the following:
docker run -d --name appname_db -e MYSQL_ROOT_PASSWORD=<password> mysql
We need a way to let our application know where this container lives (ip address) and how to connect to it (tcp?, username/password, etc). We’re in luck, with docker we can use the –link (read here for more information on how –link works) flag when spinning up our Rails app and this will inject some very useful environment variables into our app so we can reference them when our application starts. By assuming we will use the –link flag and we link our database container with the alias “appdb” (more on this later) we can change our database.yml file to look something like the following (Test/Prod config left out on purpose, see the rest here)
development:
adapter: mysql2
encoding: utf8
reconnect: false
database: appdb_dev
pool: 5
username: root
password: <%= ENV['APPDB_ENV_MYSQL_ROOT_PASSWORD'] %>
host: <%= ENV['APPDB_PORT_3306_TCP_ADDR'] %>
port: <%= ENV['APPDB_PORT_3306_TCP_PORT'] %>
Rails Container
For the ruby on rails container we can now deploy it making sure we adhere to our dynamic database information like this. Notice how we “link” this container to our “appname_db” container we ran above.
docker run -d --link appname_db:appdb -p 80:3000 --name appname wallnerryan/appname
We also map a port to 3000 because we’re running our ruby on rails application using rails server on port 3000.
Wait, let’s back track and see what we did to “containerize” our ruby on rails app. I had to show the database and configuration first so that once you see how it’s deployed it all connects, but now let’s focus on what’s actually running in the rails container now that we know it will connect to our database.
The first thing we needed to do to containerize our rails container was to create an image based on the rails implementation we had developed for ruby 1.8.7 and rails 3.2.8. These are fairly older version of the two and we had deployed on EC2 on ubuntu, so in the future we can try and use the Ruby base image but instead we will use the ubuntu:12.04 base image because this is the path of least resistance, even though we can reduce our total image size with the prior. (more about squashing our image size later in the post)
Doing this we can create Dockerfile that looks like the following. To see the code, look here We actually don’t need all these packages (I dont think) but I haven’t got around to reducing this to bare minimum by removing one by one and seeing what breaks. (This is actually easy and a fun way to get your container just right because we’re using docker, and things build and run so quickly)

As you can see we use “FROM ubuntu:12.04” to denote the base image and then we continue to install packages, COPY the app, make sure “bundler” is installed and install using the bundler the dependencies. After this we set the RAILS_ENV to use “production” and rake the assets. (We cannot rake with “db:create” because the DB does not exist at docker build time, more on this in runtime dependencies) Then we throw our init script into the container, chmod it and set it as the CMD used when the container is run via Docker run. (If some of this didn’t make sense, please take the time to run over to the Docker Docs and play with Docker a little bit. )
Great, now we have a rails application container, but a little more detail on the init script and runtime dependencies before we run this. See the below section.
Runtime dependencies:
There are a few runtime dependencies we need to be aware of when running the app in this manner, the first is that we cannot “rake db:create” until we know the database is actually running and can be connected to. So in order to make this happen at runtime we place this inside the init script.
The other portion of the runtime dependencies is to make sure that “rake db:create” does not fire off before the database is initialized and ready to use. We will use Docker Compose to deploy this app and while compose allows us to supply dependencies in the form of links there no real control over this if A) they aren’t linked, and B) if there is a time sequence needed. In this case it takes the MySQL container about 10 seconds to initialize so we need to put a “sleep 15” in our init script before firing off “rake db:create” and then running the server.
In the below script you can see how this is implemented.

Nothing special, but this ensures our app runs smoothly every time.
Running the application
We can run the app a few different ways, below we can see via Docker CLI and via Docker Compose.
Docker CLI
docker run -d --name appname_db -e MYSQL_ROOT_PASSWORD=root mysql
docker run -d --link appname_db:appdb -p 3000:3000 --name appname app_image
Docker Compose
With compose we can create a docker-comose.yml like the following.
app:
image: wallnerryan/app
cpu_shares: 100
mem_limit: 117964800
ports:
- "80:3000"
links:
- app_mysql:appdb
app_mysql:
image: mysql
cpu_shares: 100
mem_limit: 117964800
environment:
MYSQL_ROOT_PASSWORD: XXXXXX
Then run “docker compose up”
Running the app in ECS and moving DNS:
We can run this in Amazon ECS (Elastic Container Service) as well, using the same images and docker compose file we just created. If you’re unfamiliar with EC2 or the Container Service, check out the getting started guide.
First you will need the ecs cli installed, and the first command will be to setup the credentials and the ECS cluster name.
ecs-cli configure --region us-east-1 --access-key <XXXXX> --secret-key <XXXXXXXXX> --cluster ecs-cli-dev
Next, you will want to create the cluster. We only create a cluster of size=1 because we don’t need multiple nodes for failover and we aren’t running a load balancer for scale in this example, but these are all very good ideas to implement for your actual microservice application in production so you do not need to update your domain to point to different ECS cluster instances when your microservices application moves around.
ecs-cli up --keypair keypair-name --capability-iam --size 1 --instance-type t2.micro
After this, we can send our docker compose YAML file to ecs-cli to deploy our app.
ecs-cli compose --file docker-compose.yml up
To see the running app, run the following command.
ecs-cli ps
NOTE: When migrating this from EC2 make sure and update the DNS Zone File of your domain name to point at the ECS Cluster Instance.
Finally, now that the application is running
Let’s back track and squash our image size for the rails app.
There are a number of different ways we can go about shrinking our application such as a different base image, removing unneeded libraries, running apt-get remove and autoclean and a number of others. Some of these taking more effort than others, such as if we change the base image, we would need to make sure our Dockerfile still installs the needed version of gems, and we can alternatively use a ruby base image but the ones I looked at don’t go back to 1.8.7.
The method we use as a “quick squash” is to export, and import the docker image and re-label it, this will squash out images into one single image and re-import the image.
docker export 7c7e6a6fff3b | docker import - wallnerryan/appname:imported
As you can see, this squashed our image down from 256MB to 184MB, not bad for something so simple. Now I can do more, but this image size for my needs is plenty small. Here is a good post from Brian DeHamer on some other things to consider when optimizing image sizes. Below you can see the snapshot of the docker image (taxmatters is the name of the company, I have been substituting this with “appname” in the examples above).

Development workflow going forward:
So after we finished migrating to a Docker/ECS based deployment it is very easy to make changes, test in either a ECS development cluster or using Local Docker Machine, then deploy to the production closer on ECS when everything checks out. We could also imagine code changes automated in a CI pipeline where a CI pipeline kicks off lambda deployments to development after initial smoke tests triggered by git push, but we’ll leave that for “next steps” :).
Thanks for reading, cheers!
Ryan